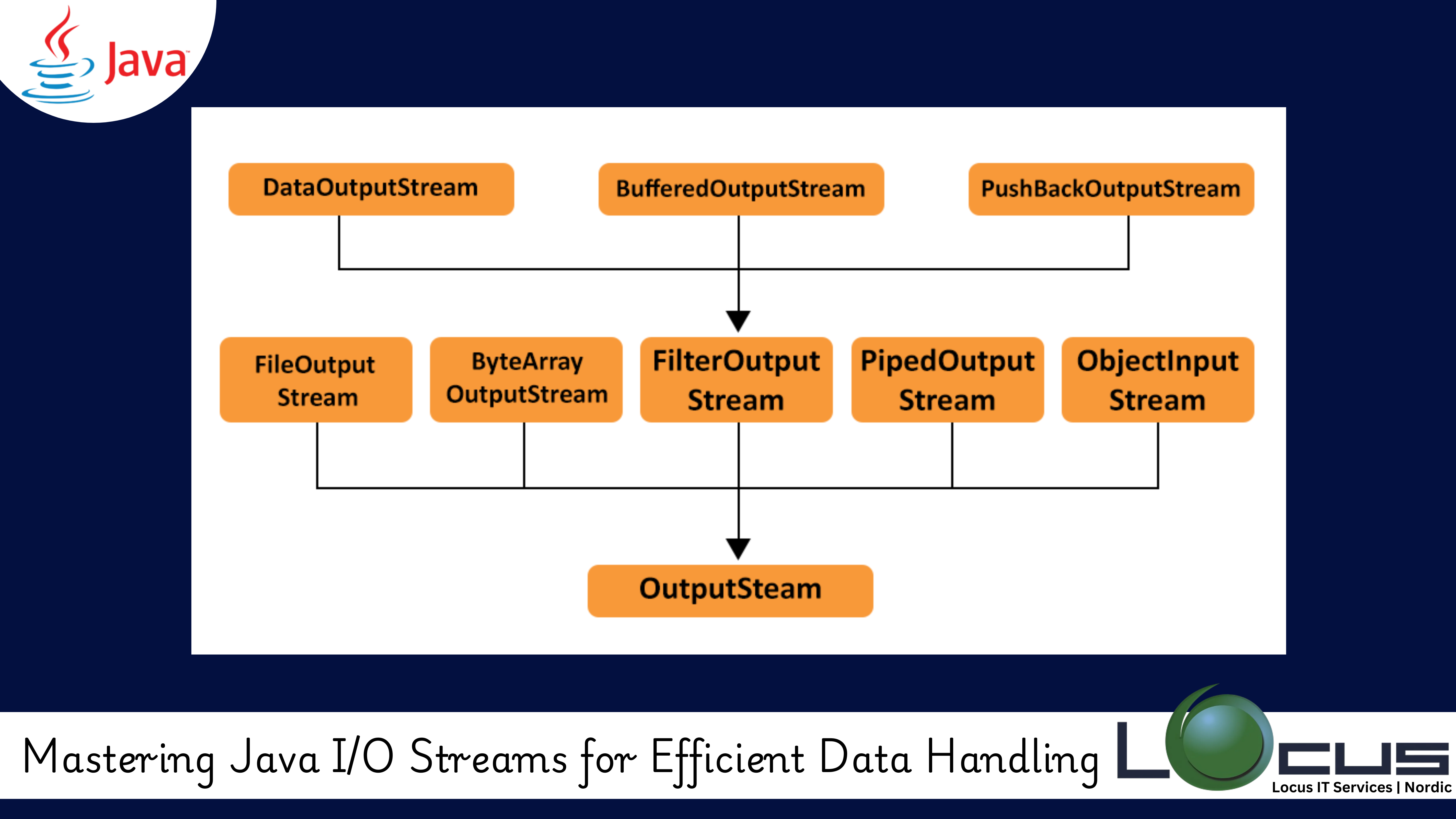
For Every Business, Data handling is at the core of many software applications, whether it’s reading configuration files, processing user input, or storing large datasets. Java, with its robust Input/Output (I/O) stream framework, offers powerful tools for working with data. This blog introduces Java I/O streams, their types, and how they can be leveraged for efficient data handling.
What Are Java I/O Streams?
In Java, I/O streams are used to read data from and write data to various sources like files, network connections, and memory buffers. The concept of a stream refers to the flow of data, which can be either:
- Input Stream: Reads data from a source into the program.
- Output Stream: Writes data from the program to a destination.
Java’s I/O streams are part of the java.io
package and are designed to handle data in a sequential, efficient, and platform-independent manner. (Ref: Installing Java Development Kit (JDK): A Step-by-Step Guide)
Types of Java I/O Streams
Java categorizes I/O streams into byte streams and character streams, based on the type of data being handled.
1. Byte Streams
Byte streams handle raw binary data. They are suitable for working with non-text data like images, audio files, or any other binary content.
- Classes:
InputStream
andOutputStream
are the parent classes for all byte streams. - Examples:
FileInputStream
andFileOutputStream
for file handling.BufferedInputStream
andBufferedOutputStream
for efficient data processing.
2. Character Streams
Character streams handle text data and ensure proper encoding and decoding of characters. They are useful for handling files or network data in human-readable formats.
- Classes:
Reader
andWriter
are the parent classes for all character streams. - Examples:
FileReader
andFileWriter
for text files.BufferedReader
andBufferedWriter
for efficient text processing.
Key Concepts in Java I/O Streams
1. Buffered Streams
Buffered streams enhance performance by reducing the number of I/O operations. They temporarily store data in memory before reading or writing it.
- Use
BufferedReader
andBufferedWriter
for text data. - Use
BufferedInputStream
andBufferedOutputStream
for binary data.
2. Chaining Streams
Java allows chaining streams to combine functionalities. For example, you can use a FileInputStream
with a BufferedInputStream
to read files efficiently.

3. Standard Streams
Java provides predefined streams for interacting with the console:
System.in
: Reads user input.System.out
: Outputs data to the console.System.err
: Outputs error messages.
4. Serialization
Serialization converts objects into a byte stream for storage or transmission. This is particularly useful for saving application state or transmitting data over networks.

Common Use Cases for Java I/O Streams
- Reading and Writing Files
- Use
FileReader
andFileWriter
for text files. - Use
FileInputStream
andFileOutputStream
for binary files.
- Use
- Processing Large Data
- Use buffered streams to handle large files efficiently.
- Network Communication
- Combine I/O streams with sockets (
Socket.getInputStream()
andSocket.getOutputStream()
) for data transmission.
- Combine I/O streams with sockets (
- Logging and Error Handling
- Redirect logs and errors to files using
PrintStream
orFileWriter
.
- Redirect logs and errors to files using
Best Practices for Working with Java I/O Streams
- Use Buffered Streams
Always use buffered streams when handling large files or frequent I/O operations to improve performance. - Close Streams Properly
Ensure streams are closed to release system resources. Use the try-with-resources statement for automatic resource management. - Handle Exceptions
Use proper exception handling to manage I/O errors, such as file not found or read/write issues. - Choose the Right Stream
Select byte streams for binary data and character streams for text data to avoid encoding issues. - Avoid Blocking I/O
For real-time or high-performance applications, consider non-blocking I/O using thejava.nio
package.
Final Thoughts
Java I/O streams provide a versatile and efficient framework for data handling across a wide range of applications. By understanding the different types of streams and their use cases, developers can process data effectively, whether it’s reading a simple text file, managing large datasets, or building robust network applications.
Mastering Java I/O streams is an essential skill for any developer aiming to build efficient, scalable, and reliable applications in today’s data-driven world. (Ref: Locus IT Services)