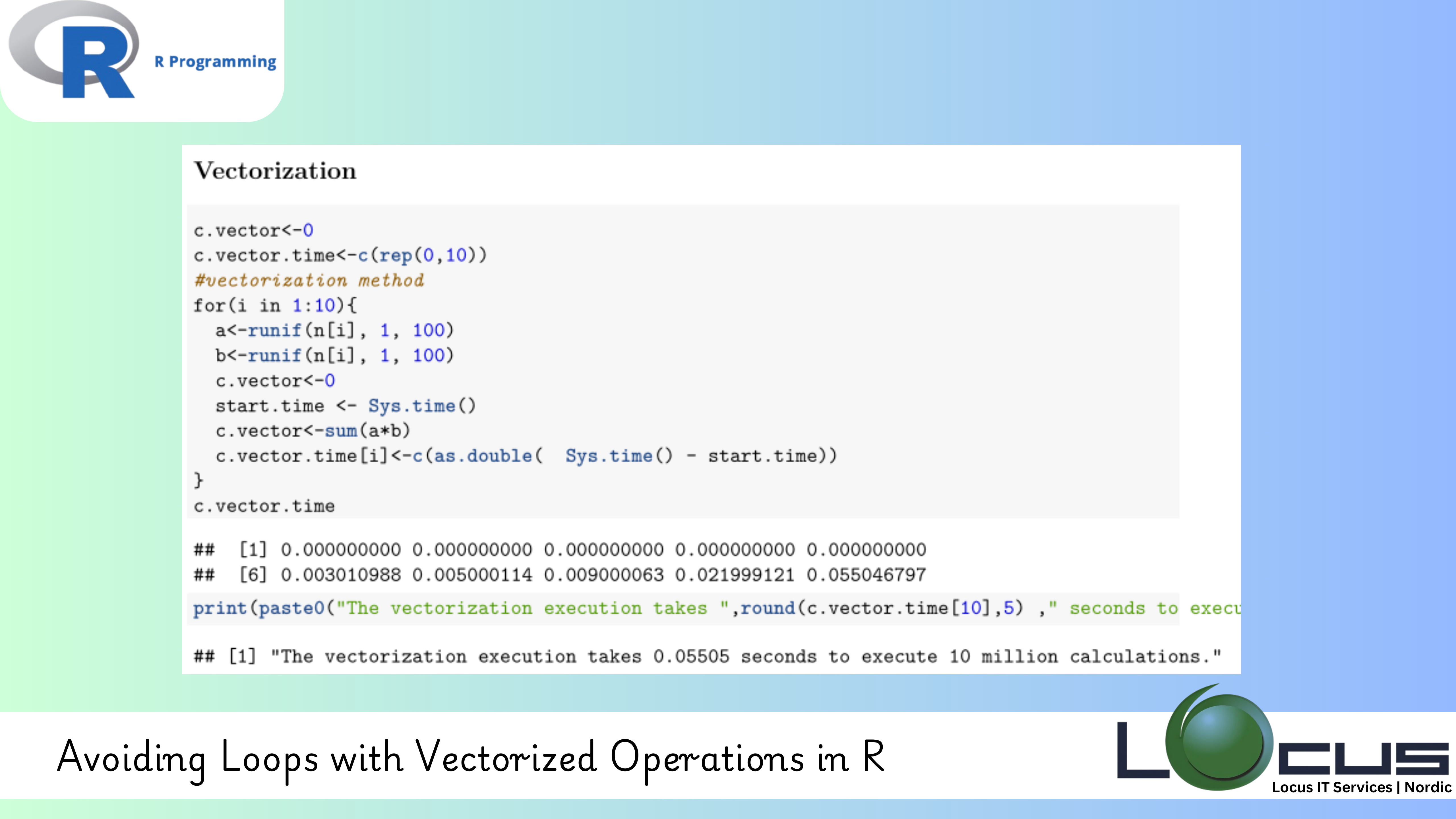
For Every Business, In R programming, Vectorized Operations in R loops are often used for repetitive tasks, such as applying a function to each element in a dataset. However, while loops can be intuitive and easy to implement, they are not the most efficient way to perform data operations in R. This is especially true when working with large datasets, where loops can be slow and memory-intensive.
The good news is that R is optimized for Vectorized Operations in R—which allow you to perform operations on entire datasets at once, without the need for explicit loops. Vectorization is a powerful feature in R that makes your code run faster, cleaner, and more efficient.
In this blog post, we’ll explore the advantages of Vectorized Operations in R and how you can avoid loops to speed up your R code. We will also show how to use vectorized functions from base R and libraries like dplyr
and purrr
to streamline your data manipulation tasks. (Ref: Working with Built-in R Functions for Seamless Data Analysis)
Why Vectorization is Better Than Loops in R
1. Speed and Efficiency
R is designed to operate on entire vectors, matrices, and data frames at once. When you use vectorized operations, R processes the data in native C code, which is much faster than processing data element by element through loops.
2. Less Memory Usage
Loops create intermediate copies of objects at each iteration, which can consume a lot of memory, especially with large datasets. Vectorized operations avoid this by operating on entire objects without creating copies.
3. Cleaner, More Readable Code
Using vectorized operations makes your code more concise and easier to understand. It reduces the need for cumbersome for
or while
loops, leading to cleaner, more readable scripts.
How to Avoid Loops with Vectorized Operations
1. Basic Arithmetic Operations
Instead of using a loop to perform an operation on each element of a vector, you can directly apply the operation to the entire vector.
Loop Example:
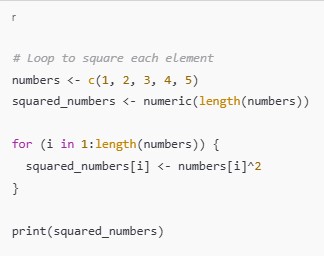
Vectorized Example:
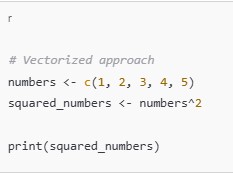
- Why this is better: The Vectorized Operations in R approach directly applies the operation on the entire vector without the need for looping through each element.
2. Using apply()
Functions for Matrices and Data Frames
If you’re working with a matrix or data frame, the apply()
function can help you avoid loops. It applies a function to the rows or columns of a matrix or data frame, providing a concise, efficient way to perform operations.
Loop Example:
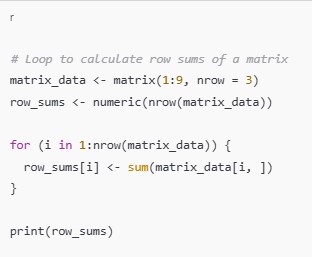
Vectorized Example with apply()
:
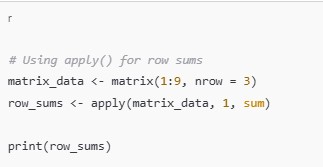
- Why this is better: The
apply()
function is more efficient and concise than manually looping through the rows.
3. Using dplyr
for Data Wrangling
For data manipulation tasks such as filtering, mutating, and summarizing data, dplyr
provides a set of highly optimized functions that can replace loops.
Loop Example (Filtering Rows):
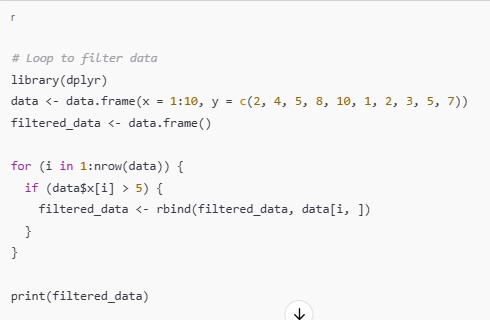
Vectorized Example with dplyr
:
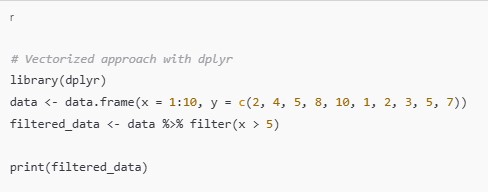
- Why this is better: The
filter()
function fromdplyr
performs the operation on the entire dataset at once, without the need for looping through rows.
4. Using purrr
for List Operations
The purrr
package is part of the Tidyverse and provides a set of functions for functional programming. It is particularly useful for applying functions over lists or vectors in a concise, efficient manner.
Loop Example:
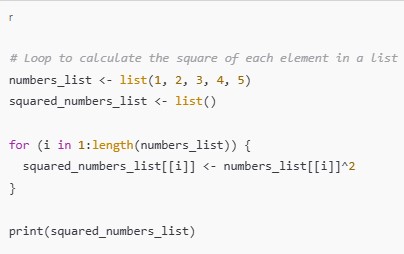
Vectorized Example with purrr
:
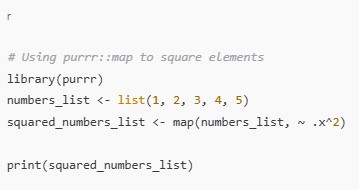
- Why this is better:
purrr::map()
is designed for this kind of task, and it internally uses Vectorized Operations in R, making the code more efficient and concise.
5. Vectorized String Operations with stringr
Another common use case where you might think of using loops is when working with strings. The stringr
package offers vectorized functions for string manipulation, making it easier to work with character data.
- Why this is better:
stringr
handles the Vectorized Operations in R string operations internally, offering a simple and efficient way to manipulate strings.
6. Making Use of R’s Rich Ecosystem
By leveraging vectorized functions from R’s extensive ecosystem of libraries—such as dplyr
, purrr
, data.table
, and others—you can take advantage of highly optimized, pre-built functions that have been tested and refined by the R community. Vectorized Operations in R This allows you to focus on higher-level tasks without worrying about the underlying implementation details.
7. Improving Reproducibility
Since vectorized code is more concise and easier to understand, it’s also more reproducible. If your code is simple and easy to follow, others (or even your future self) will be able to modify or adapt it without difficulty. This is essential for maintaining good practices in collaborative data science projects.
8. Parallelization Potential
Vectorized Operations in R lend themselves well to parallel processing. Libraries such as parallel
or future
allow you to distribute the workload across multiple processors, taking full advantage of modern multi-core processors. This is especially useful when performing large-scale data analysis or computations on big data, where a loop-based approach would be too slow to run efficiently on a single core.
9. Built-In Function Optimization
Many Vectorized Operations in R are implemented in optimized C or Fortran code under the hood, meaning they are executed much faster than custom-written loop-based equivalents. These Vectorized Operations in R are fine-tuned to make use of R’s internal data structures and minimize overhead, providing significant performance gains.
10. Avoiding Errors
With loops, especially those involving complex logic, it’s easy to make mistakes—like incorrect indexing, off-by-one errors, or forgetting to initialize variables. Vectorized Operations in R functions abstract away such complexities, reducing the risk of errors and making your code easier to debug.
Final Thoughts:
In Vectorized Operations in R are a powerful tool that can significantly improve the performance, readability, and efficiency of your code. By avoiding loops and using functions like those in dplyr
, purrr
, and stringr
, you can perform complex data manipulations and analysis tasks much faster.
When you embrace Vectorized Operations in R, your R code will be more concise, your processing time will be reduced, and your memory usage will be optimized—especially when working with large datasets. So, next time you’re tempted to use a loop in R, ask yourself: “Can I make this Vectorized Operations in R instead?” You’ll be amazed at how much faster and cleaner your code can become! (Ref: Locus IT Services)