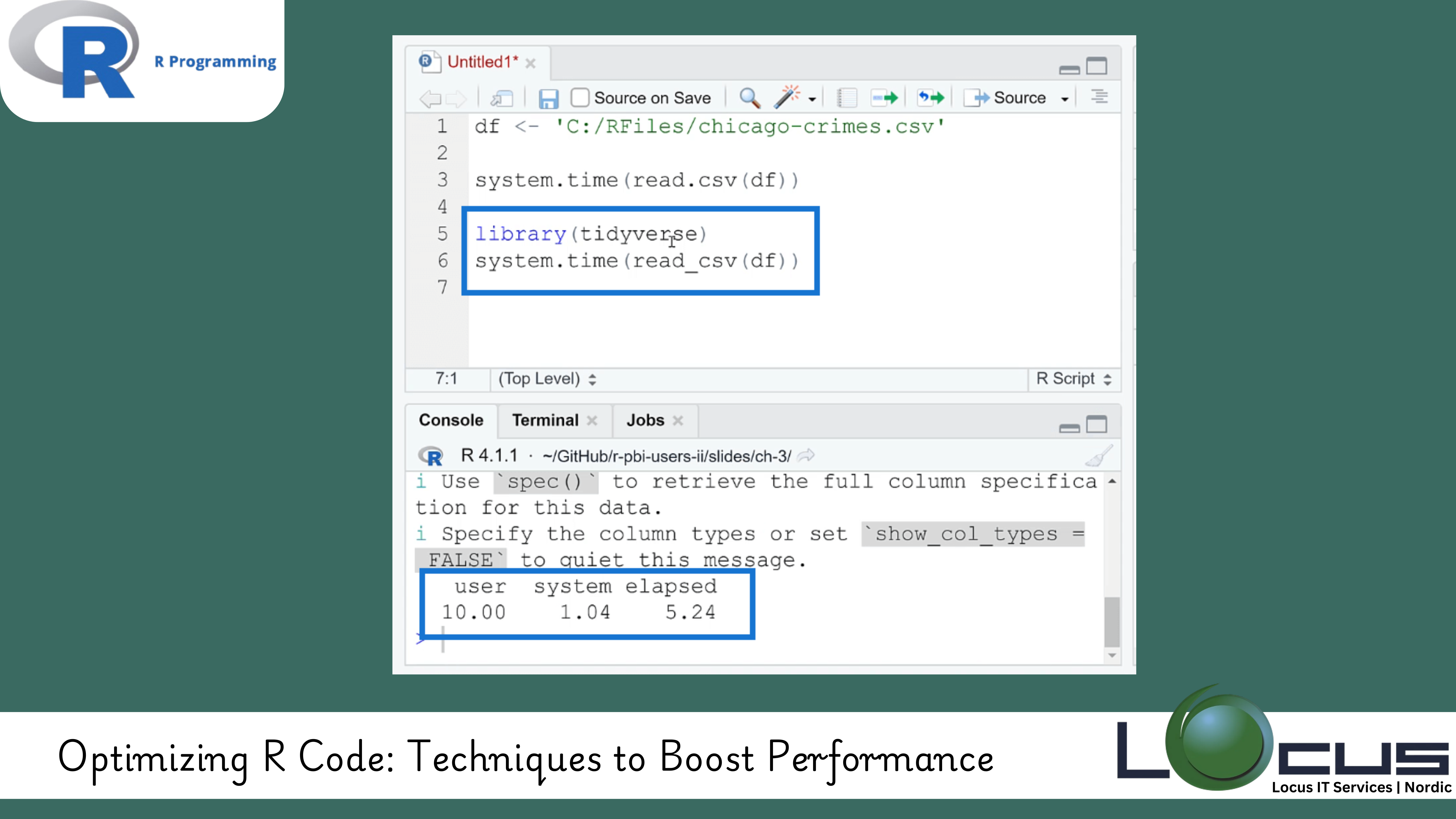
For Every Business, R is a powerful language for data analysis, statistical computing, and visualization. However, as datasets grow larger and analyses become more complex, poorly optimized R code can lead to slow execution times, excessive memory usage, and frustration. Fortunately, with a few best practices and optimization techniques, you can significantly improve the performance and efficiency of your R scripts.
In this blog post, we’ll explore key strategies for optimizing R code to ensure faster execution and better scalability.
Why Optimize R Code?
Optimizing R code is essential for improving the performance and efficiency of your analysis, especially as the complexity of your datasets and models grows. Here are the key reasons why optimizing R code is important:
1. Performance Gains
Optimized code runs faster and is more efficient. This is particularly critical when working with large datasets or running complex statistical models.
- Faster Execution: Unoptimized code, such as using loops for element-wise operations or repeatedly calculating the same values, can slow down the execution. By optimizing the code (e.g., through vectorized operations, applying efficient built-in functions), execution time can be significantly reduced.
- Time Saving: Faster code means you can process large datasets or run more complex analyses in a shorter amount of time, allowing you to make quicker decisions or iterate on models more rapidly.
For example, vectorized operations in R (which work on entire arrays or vectors at once) are much faster than using for-loops to iterate through individual elements. (Ref: Automating Data Reporting with R Functions)
2. Resource Efficiency
Optimizing R code also leads to better resource efficiency, meaning that it minimizes memory and CPU usage.
- Memory Usage: When working with large datasets, inefficient code may create unnecessary copies of data or use memory poorly. This can lead to memory overflow or slowdowns. Optimized code minimizes memory usage by handling large objects more effectively and avoiding copying data unnecessarily.
- CPU Usage: Efficient code utilizes the CPU more effectively, executing tasks with fewer computational resources, freeing up the processor for other tasks. By reducing redundant computations and optimizing data storage, your system can handle more complex operations without overloading.
For instance, instead of repeatedly reading or writing large datasets to disk, optimized code can keep data in memory and perform operations in place.
3. Scalability
As your datasets grow or your analysis becomes more complex, scalability becomes a crucial factor. Well-optimized R code is capable of handling larger datasets and more complex tasks without crashing or stalling.
- Handling Big Data: When dealing with big data, inefficient code can cause your machine to run out of memory, or the process might take too long to finish. Optimized code allows you to scale your analysis to larger datasets, ensuring that your methods remain effective even as data volumes increase.
- Complex Models: As you build more sophisticated models, such as machine learning algorithms or simulations, optimized code ensures that these models can run without delays or resource exhaustion, even when they become computationally intensive.
Optimizing your algorithms, for example, by using parallel computing or distributed processing, can enable you to handle tasks that would otherwise be impractical with standard methods.
4. Maintainability
Optimized code is cleaner and easier to maintain over time.
- Readability: Well-optimized code is often simpler and more concise. This makes it easier for you (or others) to understand, debug, and modify the code in the future. For instance, using vectorized functions instead of complex loops makes the code more readable and intuitive.
- Debugging: Code that is well-structured and optimized is easier to debug. You’ll face fewer edge cases, fewer bugs related to memory usage or resource allocation, and fewer performance issues. This means less time spent troubleshooting and more time spent analyzing and improving your models.
- Long-Term Efficiency: As projects grow, code can become difficult to manage if it’s not optimized. Optimized code ensures that future updates or extensions to your analysis are handled smoothly and efficiently, preventing bottlenecks from appearing later in the project.
Techniques for Optimizing R Code
1. Use Vectorized Operations Instead of Loops
R is designed to operate on entire vectors, matrices, or arrays in one step, making vectorized operations faster than loops. For example:
- Avoid: Loops for element-wise operations.
- Use Instead: Built-in vectorized functions like
rowSums()
,mean()
, orapply()
.
Example:
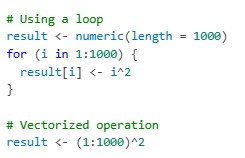
2. Leverage Built-in Functions
R’s built-in functions are written in optimized C or Fortran code, making them much faster than custom R loops or functions. Always prefer these functions for tasks like sorting, aggregating, or statistical calculations.
Example:
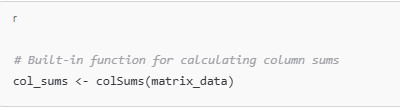
3. Avoid Growing Objects Inside Loops
Expanding vectors or data frames inside a loop is computationally expensive because R repeatedly reallocates memory. Pre-allocate the size of the object instead.
Example:
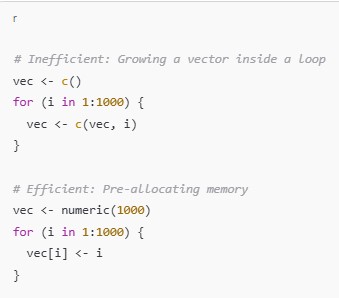
4. Use the Data Table for Faster Data Manipulation
The data.table
package is highly optimized for large datasets and provides faster data manipulation capabilities compared to base R.
Example:
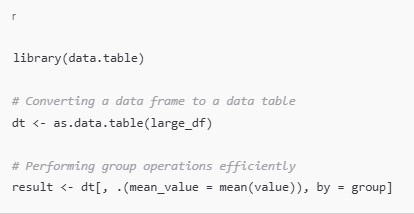
5. Profile Your Code
Use R’s built-in profiling tools like profvis
or Rprof
to identify bottlenecks in your code. Optimizing R code These tools help you understand which parts of your code consume the most time and resources, allowing you to focus your optimization efforts.
Example:
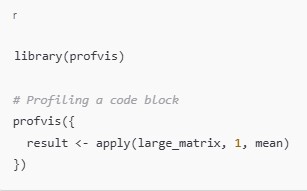
6. Minimize Data Copying
R uses copy-on-modify semantics, meaning modifying an object often results in a copy being created. Optimizing R code To avoid unnecessary copies, work with data efficiently, and avoid repeatedly modifying large objects.
7. Use Efficient Libraries
Third-party libraries often provide faster alternatives to base R functions. For example:
dplyr
: For efficient data manipulation with a readable syntax.data.table
: For handling large datasets with speed.Matrix
: For working with sparse and dense matrices efficiently.
8. Avoid Nested Loops
Nested loops can significantly slow down execution. Optimizing R code Where possible, use vectorized or apply-family functions (lapply()
, sapply()
, etc.) instead.
Example:
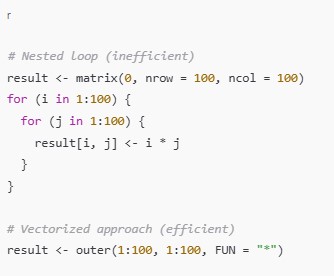
9. Parallelize Your Work
Take advantage of multiple cores on your machine using parallel computing libraries like parallel
, future
, or foreach
. This is especially useful for tasks like simulations, bootstrapping, and large-scale data processing.
Example:
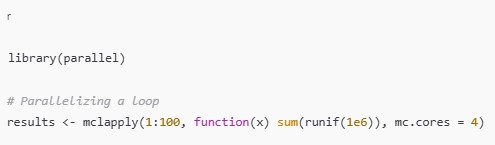
10. Avoid Repeated Computations
If a value or result is used multiple times, calculate it once and store it instead of recalculating it repeatedly.
Example:
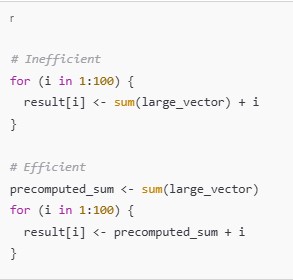
Additional Tips
- Use Logical Indexing: Instead of loops for filtering, use logical vectors.
- Clean Your Environment: Avoid storing large, unused objects in memory.
- Use Lazy Evaluation: Functions in R use lazy evaluation, so only calculate what’s needed.
- Reduce Disk I/O: Minimize reading/writing data repeatedly; load data once and keep it in memory.
Final Thoughts
Optimizing R code is a blend of understanding the language’s strengths, leveraging efficient tools, and following best practices. By focusing on techniques such as vectorization, parallelization, and using optimized libraries, you can write faster and more efficient R scripts.
Whether you’re processing massive datasets, running simulations, or building machine learning models, these Optimizing R code strategies will help you unlock the full potential of R. Start implementing these techniques in your workflows today and see the difference in performance! (Ref: Locus IT Services)