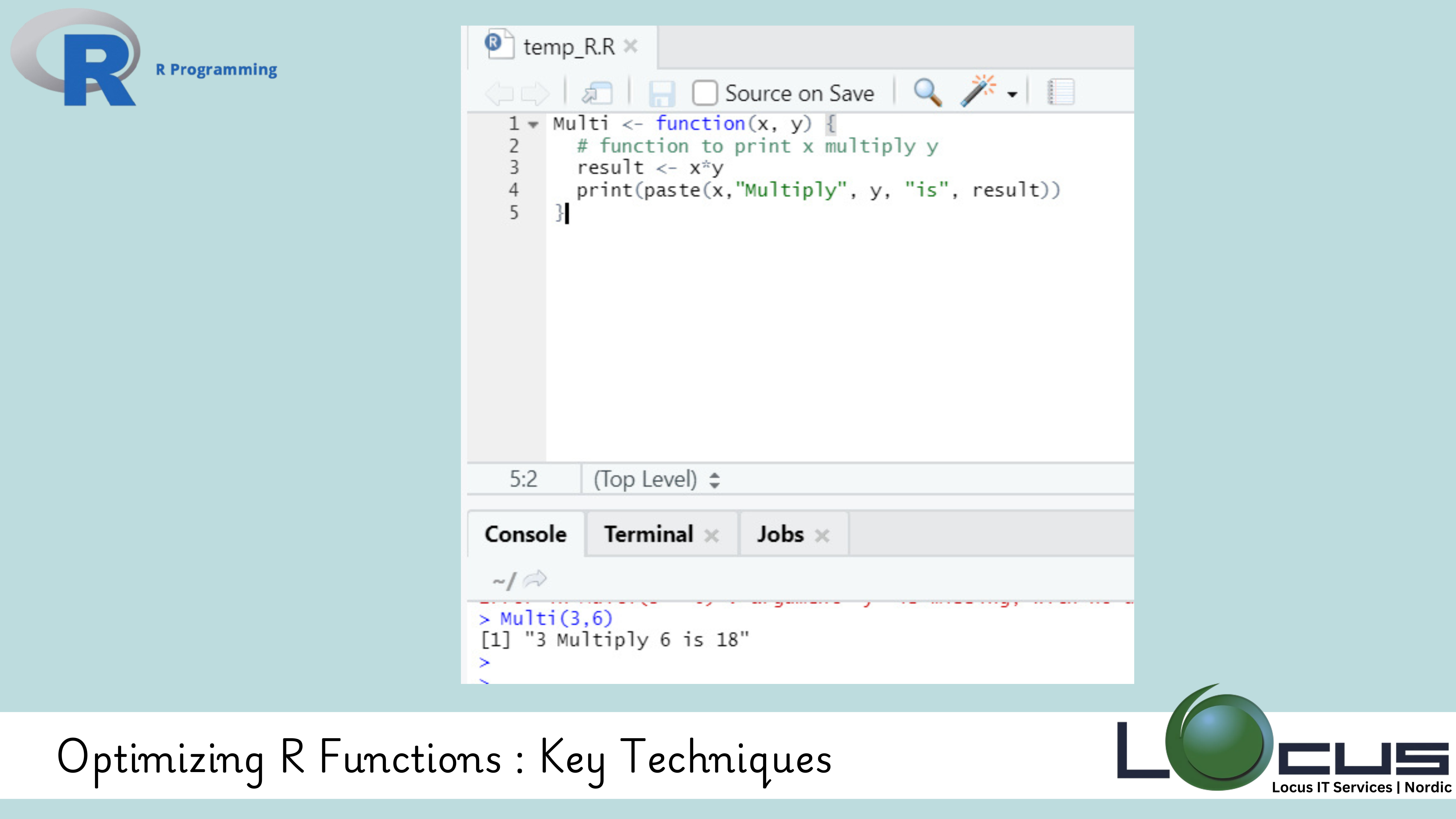
For Every Business, efficiency and precision are paramount. As datasets grow in complexity, leveraging the power of R functions becomes essential for any data analyst or scientist. Functions in R are more than just blocks of reusable code; they are the building blocks of sophisticated analysis pipelines. This blog post delves into the fundamentals, advanced techniques, and best practices for mastering R functions to supercharge your data analysis workflow.
Why Functions Are Crucial in R
Functions are the backbone of programming in R. They allow you to: (Ref: R Programming for Data Analysis)
- Automate repetitive tasks: Save time and minimize errors by encapsulating complex operations into reusable units.
- Enhance readability: Break down complex analyses into manageable, well-defined steps.
- Ensure reusability: Share and reuse code across projects, ensuring consistency and reducing redundancy.
Writing Your First R Function
Creating a function in R is straightforward. The basic syntax involves defining the function name, arguments, and the code block:
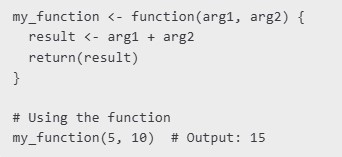
In this simple example, the function takes two arguments, performs an addition, and returns the result.
Advanced Techniques for R Functions
Once you’re comfortable with basic functions, it’s time to explore advanced features:
1. Default Arguments
Default arguments make functions more flexible:
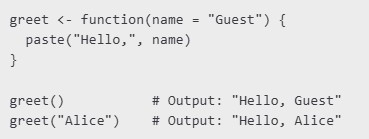
2. Anonymous Functions
Anonymous functions are useful for one-off operations, especially with apply-family functions:
sapply(1:5, function(x) x^2) # Output: [1, 4, 9, 16, 25]
3. Vectorization
R excels in vectorized operations, allowing functions to handle entire vectors at once without explicit loops:
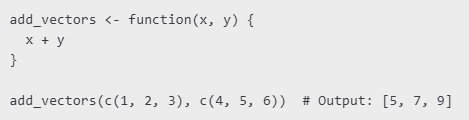
Debugging and Optimizing Functions
Effective debugging and optimization can significantly enhance performance:
- Debugging Tools: Use
debug()
,traceback()
, andbrowser()
to inspect your code. - Profiling: Identify bottlenecks with
Rprof
or theprofvis
package. - Best Practices: Write concise functions that focus on a single task. Avoid excessive nesting to maintain readability.
Integrating Functions with the Tidyverse
The Tidyverse ecosystem makes it easy to combine functional programming with data manipulation:
Example: Using Purrr for Functional Programming
library(purrr)
numbers <- list(1:3, 4:6, 7:9)
map(numbers, sum) # Output: [6, 15, 24]
By using purrr
, you can apply functions to lists and other complex structures efficiently.
Real-World Applications
Mastering R functions opens the door to solving real-world problems, such as:
- Exploratory Data Analysis (EDA): Automate repetitive summarization tasks.
- Custom Pipelines: Build end-to-end analysis pipelines tailored to specific projects.
- Reporting: Generate automated reports with parameterized functions.
Best Practices for Mastering R Functions
1. Document Your Functions: Use Roxygen2 to Create Detailed Documentation
Documentation is key to writing maintainable and reusable code. Roxygen2 is a powerful tool in R for documenting your functions effectively. It allows you to add detailed comments directly above your function definitions, which can then be converted into help files. These help files provide clear instructions for anyone using the function, including its purpose, inputs, outputs, and any dependencies.
By using Roxygen2, you ensure that your functions are well-documented, making them easier to understand and use for others (or even yourself after some time).
2. Test Thoroughly: Write Unit Tests with testthat
to Ensure Your Functions Work as Expected
Testing is crucial for ensuring the reliability of your functions. The testthat
package in R provides a framework for creating unit tests, which help verify that your functions perform as intended under various conditions. Writing tests ensures that your code produces the expected output and reduces the risk of bugs.
By running these tests regularly, especially after making changes to your code, you can ensure that your functions remain robust and error-free.
3. Keep It Modular: Break Down Large Tasks into Smaller, Focused Functions
Complex tasks can often be overwhelming to manage within a single function. By breaking them into smaller, modular functions, you make your code easier to read, debug, and reuse. Modular functions focus on a single responsibility, which also makes them easier to test and optimize.
Example: Imagine you need to analyze a dataset by cleaning it, performing statistical calculations, and visualizing the results. Instead of creating one large function, you can break it into three modular functions:
This approach makes your code more organized and adaptable to future changes. If one part of the analysis needs to be updated, you can modify just the relevant function without impacting the rest of the workflow.
Final Thoughts
Mastering R functions is a game-changer for effective data analysis. From basic operations to advanced functional programming, the power of R functions lies in their ability to streamline workflows, improve code quality, and deliver actionable insights. Start practicing today, and watch your data analysis skills soar to new heights.
Are you ready to elevate your R programming journey? Let us know your favorite use cases for R functions in the comments below! (Ref: Locus IT Services)