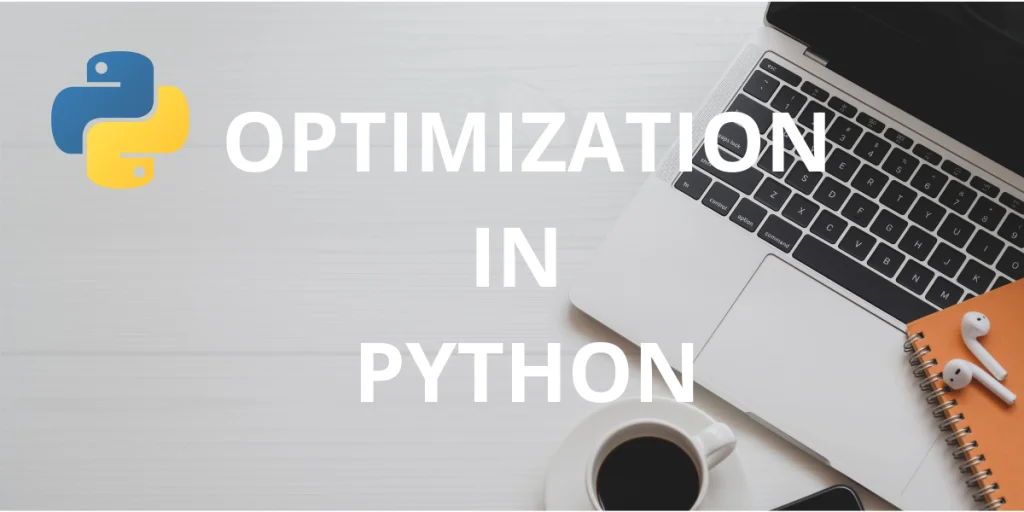
For Every Business programming, developing efficient algorithms is as critical as crafting the solution itself. Poorly optimized algorithms can lead to sluggish performance, resource bottlenecks, and even application failure in large-scale systems. Python, known for its simplicity and versatility, provides an extensive suite of tools and libraries to optimize algorithms effectively.
In this blog, we’ll explore how Python empowers developers to optimize algorithms, enhance performance, and solve complex computational problems seamlessly.
What is Algorithm Optimization?
Algorithm optimization refers to the process of improving an algorithm’s efficiency in terms of speed, memory usage, or scalability. This involves refining the logic, employing better data structures, or leveraging specialized techniques to achieve optimal performance. (Ref: Python Regression Models: Transform Predictive Insights)
Key goals of optimization include:
- Reducing Time Complexity: Lowering the time it takes to execute.
- Reducing Space Complexity: Minimizing memory usage.
- Improving Scalability: Ensuring performance remains robust as data sizes grow.
Why Python for Algorithm Optimization?
While Python is often considered slower than compiled languages like C++ or Java, its extensive ecosystem and adaptability make it a powerhouse for algorithm optimization.
- Rich Libraries: Python boasts a wide array of libraries, such as NumPy, SciPy, and Pandas, that are optimized for computational tasks.
- Ease of Implementation: Python’s syntax and readability allow developers to focus on refining algorithms rather than debugging low-level details.
- Integration with Faster Code: Tools like Cython and Numba enable Python to integrate with C-level performance for critical parts of the algorithm.
- Versatile Tools: Profiling tools like
cProfile
andline_profiler
help identify bottlenecks in the code. - Parallelism and Concurrency: Libraries like multiprocessing, threading, and Dask make it easier to implement parallel computations.
Strategies for Optimizing Algorithms in Python
1. Profiling and Identifying Bottlenecks
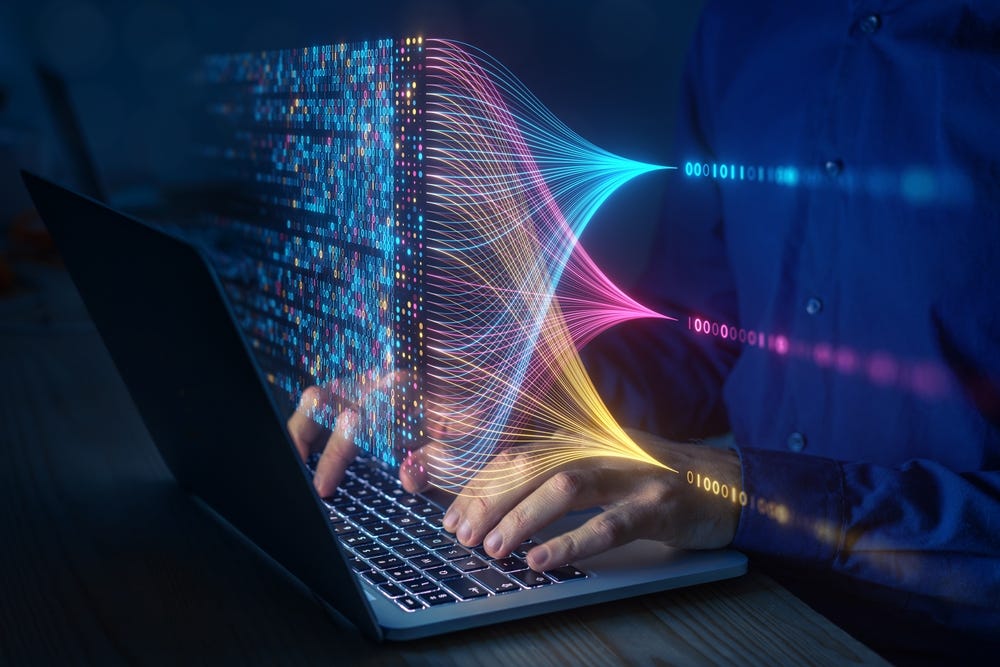
Before algorithm optimization, it’s crucial to identify which parts of your code are slowing things down. Python offers robust profiling tools:
- cProfile: A built-in library that provides detailed insights into function call times.
- line_profiler: Breaks down execution times line-by-line to pinpoint inefficiencies.
2. Choosing the Right Data Structures
The choice of data structures has a significant impact on algorithm performance.
- Use lists for dynamic arrays, but prefer NumPy arrays for numerical computations.
- Employ dictionaries for fast key-value lookups.
- Opt for sets when checking membership in large collections.
3. Optimizing Loops
Nested loops or inefficient looping structures often lead to slow performance.
- Replace loops with vectorized operations using NumPy.
- Use Python’s built-in functions like
map()
,filter()
, or comprehensions for concise and faster iterations.
4. Leveraging Built-in Libraries
Python’s standard and third-party libraries are highly optimized for specific tasks.
- Use math for mathematical computations instead of implementing from scratch.
- Rely on NumPy for matrix operations and numerical calculations.
- Utilize Pandas for efficient data manipulation.
5. Parallelism and Concurrency
For algorithms that can run tasks independently, parallel execution can significantly improve performance.
- Threading: Use the
threading
module for I/O-bound tasks. - Multiprocessing: Leverage multiple CPU cores for computationally heavy tasks.
- Dask: Scales parallelism for large datasets.
6. Using Just-in-Time Compilation
Libraries like Numba and PyPy optimize Python code by compiling it into machine code at runtime.
- Numba: Accelerates numerical computations with minimal changes to the Python code.
- PyPy: A high-performance alternative to CPython for long-running applications.
7. Algorithmic Improvements
Revisiting the logic itself often yields better performance gains.
- Replace brute-force approaches with dynamic programming or divide-and-conquer strategies.
- Use efficient search and sort algorithms like binary search or quicksort.
- Reduce unnecessary computations by caching results with memoization.
8. Reducing Memory Usage
Efficient memory usage is crucial for large datasets.
- Avoid creating unnecessary copies of data structures.
- Use generators instead of lists for memory-efficient iteration.
- Opt for sparse matrices in SciPy when dealing with datasets with many zeros.
Python Libraries for Algorithm Optimization
1. NumPy
- Optimized for numerical operations, matrix manipulations, and vectorized computations.
- Ideal for replacing Python loops with faster array-based calculations.
2. SciPy
- Built on NumPy, providing advanced functionality like optimization, integration, and linear algebra.
3. Cython
- Converts Python code into C for significant performance boosts.
- Especially useful for critical parts of an algorithm.
4. Numba
- A Just-in-Time (JIT) compiler that accelerates Python code execution by compiling it into machine code.
5. Dask
- Scales computations across multiple cores or distributed systems, making it perfect for big data.
6. SymPy
- Symbolic computation library useful for mathematical optimizations and simplifications.
Common Applications of Algorithm Optimization
1. Big Data Processing
Efficient algorithms are vital when working with large datasets in fields like finance, healthcare, and e-commerce. Python libraries like Dask and PySpark provide scalable solutions.
2. Machine Learning
Optimizing algorithms for feature selection, training models, or hyperparameter tuning can significantly reduce computation time and improve accuracy.
3. Scientific Simulations
Fields like physics, chemistry, and biology rely on Python for running computationally intensive simulations. Libraries like NumPy and SciPy are crucial here.
4. Real-Time Systems
From autonomous vehicles to fraud detection, real-time applications require optimized algorithms for low-latency performance.
Challenges in Algorithm Optimization
- Balancing Complexity and Readability: Highly optimized code can become harder to read and maintain.
- Hardware Limitations: Some optimizations require advanced hardware, such as GPUs or multi-core processors.
- Dimensionality: High-dimensional data can exponentially increase computation times.
- Over-Optimization: Excessive focus on optimization can lead to diminishing returns and resource wastage.
Best Practices for Algorithm Optimization
- Start with Profiling: Focus on optimizing bottlenecks rather than prematurely optimizing the entire code.
- Test Incrementally: Measure the impact of each optimization step to avoid overcomplication.
- Maintain Code Readability: Document changes and ensure that optimized code remains understandable.
- Leverage Community Resources: Use pre-built libraries and solutions to save development time.
Final Thoughts
Python’s combination of simplicity, versatility, and a powerful ecosystem makes it an ideal choice for algorithm optimization. By leveraging its profiling tools, advanced libraries, and efficient coding techniques, developers can significantly improve algorithm performance across a wide range of applications.
Whether you’re optimizing for big data analytics, machine learning models, or real-time systems, Python provides the tools and frameworks to achieve unparalleled efficiency. Mastering these techniques not only enhances performance but also positions you to tackle increasingly complex computational challenges with confidence.