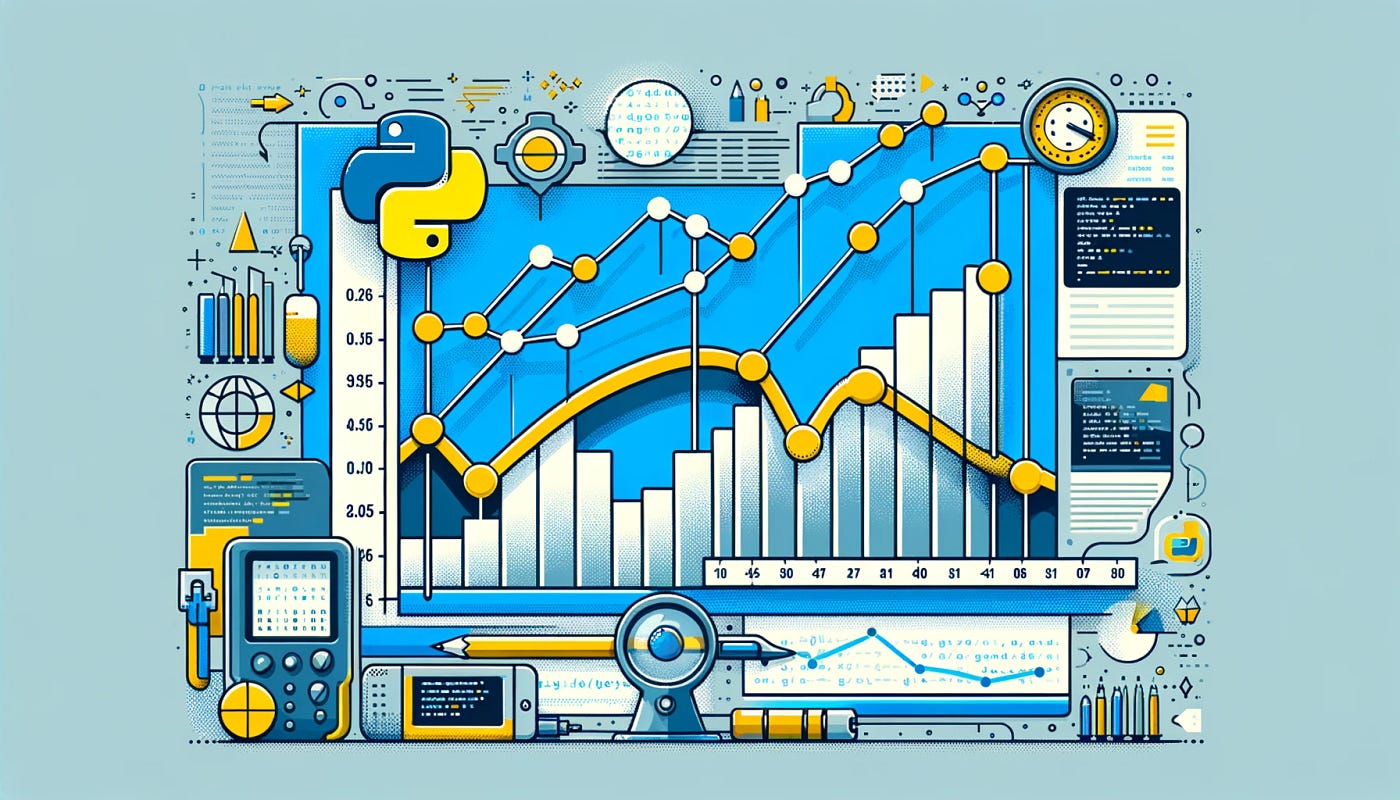
Regression modeling is a cornerstone of data analysis, enabling us to predict continuous outcomes and uncover relationships between variables. Python, with its rich ecosystem of libraries, provides powerful tools for building, evaluating, and interpreting regression models. From simple linear regression to advanced machine learning techniques, Python empowers data scientists to extract meaningful insights from data.
In this blog, we’ll explore Python regression models, their types, use cases, and essential libraries, along with best practices for implementation.
What is Regression Modeling?
Regression modeling is a statistical technique used to predict a continuous dependent variable based on one or more independent variables. It’s widely used across industries to solve problems like sales forecasting, risk assessment, and pricing optimization. (Ref: Python for Computer Vision)
Key objectives of regression modeling include:
- Prediction: Estimating future outcomes based on historical data.
- Understanding Relationships: Identifying how independent variables influence the dependent variable.
- Decision-Making: Supporting business and scientific decisions with data-driven insights.
Types of Regression Models in Python
1. Linear Regression
- Overview: The simplest form of regression models, where the relationship between the dependent and independent variables is modeled as a straight line.
- Use Cases: Predicting house prices, stock prices, or customer spend based on factors like size, time, or demographics.
- Tools in Python:
LinearRegression
from Scikit-learn,statsmodels.api.OLS
.
2. Multiple Linear Regression
- Overview: An extension of linear regression that involves multiple independent variables.
- Use Cases: Estimating sales based on advertising spend across multiple channels.
- Tools in Python: Same as linear regression with support for multivariable input.
3. Polynomial Regression
- Overview: Models a non-linear relationship by introducing polynomial terms.
- Use Cases: Capturing trends in data where linear models fall short, such as growth patterns.
- Tools in Python: Use Scikit-learn’s
PolynomialFeatures
for feature engineering.
4. Ridge and Lasso Regression
- Overview: Regularized regression techniques that reduce overfitting by penalizing large coefficients.
- Ridge Regression: Adds L2 regularization (squared magnitude of coefficients).
- Lasso Regression: Adds L1 regularization (absolute value of coefficients), also performing feature selection.
- Use Cases: Handling high-dimensional data with many predictors.
- Tools in Python:
Ridge
andLasso
classes from Scikit-learn.
5. Logistic Regression
- Overview: A regression models used for binary classification problems. While named “regression,” it predicts probabilities rather than continuous outcomes.
- Use Cases: Predicting customer churn, disease diagnosis (yes/no outcomes).
- Tools in Python:
LogisticRegression
from Scikit-learn.
6. Support Vector Regression (SVR)
- Overview: A machine learning technique that uses hyperplanes to fit the data within a margin of tolerance.
- Use Cases: Predicting non-linear trends in financial markets or weather data.
- Tools in Python:
SVR
from Scikit-learn.
7. Decision Tree and Random Forest Regression
- Overview: Tree-based algorithms that split data into branches to make predictions. Random forests are ensembles of decision trees for improved accuracy.
- Use Cases: Pricing optimization, sales forecasting with complex interactions.
- Tools in Python:
DecisionTreeRegressor
andRandomForestRegressor
from Scikit-learn.
8. Gradient Boosting Models
- Overview: Advanced tree-based models like XGBoost, LightGBM, and CatBoost that iteratively improve predictions.
- Use Cases: Predicting complex relationships in structured data, like customer lifetime value.
- Tools in Python:
XGBRegressor
,LGBMRegressor
,CatBoostRegressor
.
Popular Python Libraries for Regression
1. Scikit-learn
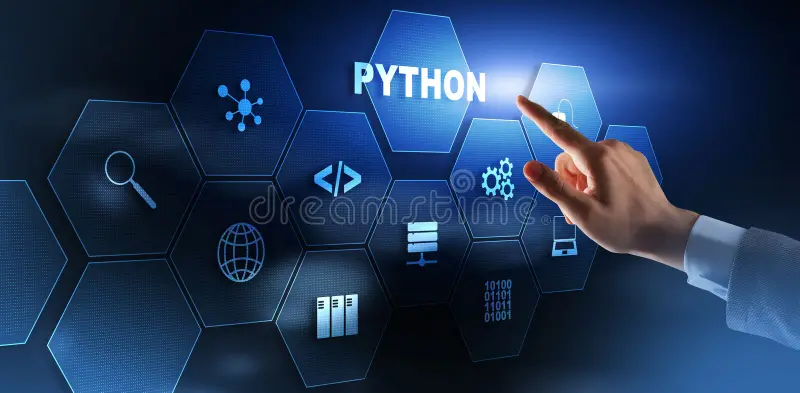
- Offers a comprehensive suite of regression models, from linear to ensemble techniques.
- Includes tools for preprocessing, feature engineering, and model evaluation.
2. Statsmodels
- Ideal for statistical analysis and hypothesis testing in regression.
- Provides detailed summaries of regression outputs, including p-values and confidence intervals.
3. TensorFlow and PyTorch
- Suitable for building custom regression models, especially in deep learning applications.
4. Pandas and NumPy
- Essential for data manipulation, cleaning, and feature engineering before modeling.
5. Matplotlib and Seaborn
- Useful for visualizing relationships, residuals, and model performance.
Steps to Build a Regression Model in Python
- Data Preparation
- Collect Data: Use structured datasets relevant to your problem.
- Clean Data: Handle missing values, outliers, and inconsistencies.
- Feature Engineering: Create, transform, or encode features to improve model performance.
- Exploratory Data Analysis (EDA)
- Use visualization tools like Seaborn to analyze variable relationships.
- Identify correlations and patterns.
- Model Selection
- Choose the appropriate regression technique based on the data and problem complexity.
- Train-Test Split
- Divide the data into training and testing sets to evaluate the model’s generalization.
- Model Training
- Fit the model to the training data using libraries like Scikit-learn.
- Evaluation
- Use metrics like Mean Absolute Error (MAE), Mean Squared Error (MSE), and R-squared to assess model performance.
- Analyze residuals to detect patterns or biases.
- Optimization
- Tune hyperparameters using techniques like grid search or random search.
- Apply regularization if overfitting occurs.
- Deployment
- Integrate the model into production using Flask, FastAPI, or cloud services.
Key Metrics for Regression Model Evaluation
- Mean Absolute Error (MAE): Measures the average absolute differences between predicted and actual values.
- Mean Squared Error (MSE): Penalizes larger errors by squaring the differences.
- Root Mean Squared Error (RMSE): Square root of MSE, providing error in the same unit as the data.
- R-squared (R²): Indicates the proportion of variance in the dependent variable explained by the model.
- Adjusted R-squared: Adjusts R² for the number of predictors, useful in multiple regression.
Common Challenges in Regression Modeling
1. Multicollinearity
- Occurs when independent variables are highly correlated.
- Solution: Remove correlated variables or use regularization techniques like Ridge or Lasso regression.
2. Overfitting
- Happens when the model performs well on training data but poorly on test data.
- Solution: Use cross-validation, regularization, or simpler models.
3. Outliers
- Extreme values can distort regression results.
- Solution: Use robust regression techniques or transform variables.
4. Non-Linearity
- Linear models may not capture non-linear relationships.
- Solution: Use polynomial regression or tree-based methods.
Applications of Regression Models
- Finance: Stock price prediction, risk modeling, and financial forecasting.
- Healthcare: Predicting patient outcomes, disease progression, and treatment costs.
- Marketing: Customer segmentation, sales forecasting, and ROI estimation.
- Energy: Demand forecasting and optimizing resource allocation.
Final Thoughts
Regression models are indispensable tools in data science, offering insights into relationships and enabling predictive capabilities. Python, with its powerful libraries and user-friendly ecosystem, makes building and deploying regression models accessible to both beginners and experts.
By understanding the types of regression models and their applications, and by following best practices, you can unlock the full potential of Python for regression analysis, driving meaningful insights and decisions across industries.